Reading from Text Files
Reading from an existing file is a very simple extension of the code example we used in Working with Text Files, except this time we will utilise the ReadLine function of the TextStream object from FileSystemObject to return a string containing the first line from our file.
Reading the First Line of Text
'open and read the contents of an existing file
Dim strFileName As String
strFileName = "C:\Temp\MyWorld.txt"
'declare the variables for use with the Scripting library
Dim fso As New Scripting.FileSystemObject
Dim fsoStream As Scripting.TextStream
'open an existing text file
Set fsoStream = fso.OpenTextFile(strFileName, ForReading)
'read a line to the file and display in a message box
With fsoStream
End With
If everything worked you will get the following message. If not, make sure that your text file exists and that it has at least one line of text.
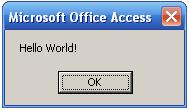
Of course in most cases we want to read more than just the first line of the file. For this we add a simple Do While…Loop and reference the property AtEndOfStream which tells us that the end of the file has been reached. Normally we would read each line and run some kind of actions on it, such as adding it to the database or checking the value against some previously stored values – feel free to add these at the TODO line.
Reading Every Line of Text
'open and read the contents of an existing file
Dim strFileName As String
strFileName = "C:\Temp\MyWorld.txt"
'declare the variables for use with the Scripting library
Dim fso As New Scripting.FileSystemObject
Dim fsoStream As Scripting.TextStream
'open an existing text file
Set fsoStream = fso.OpenTextFile(strFileName, ForReading)
'read a line to the file and display in a message box
With fsoStream
Do While Not .AtEndOfStream
'TODO: add some code here to process each line
MsgBox .ReadLine
Loop
End With